
JavaScript basics
Definition: Java is a programming language used to create websites alongside HTML and CSS. While HMTL creates the content of the website, CSS changes the design of such elements. However, JavaScript adds functionality to those elements.
JavaScript can also be used to modify HTML content.
For example, if you go to any website ( like https://www.amazon.com/) and then click on inspect.
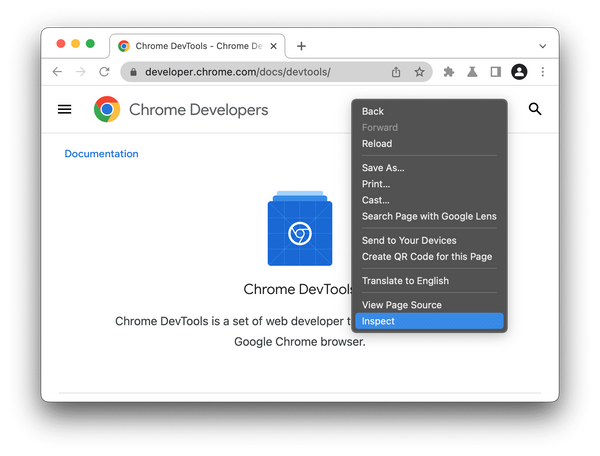
And after that, click on “console” and type: document.body.innerHTML = 'hello';
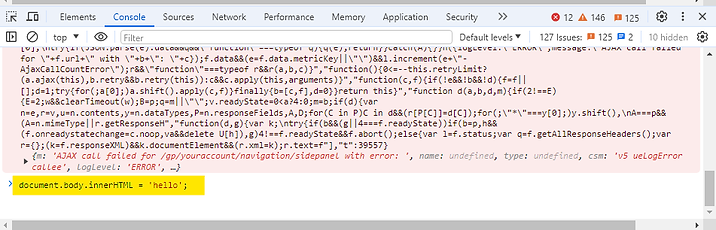
JavaScript will replace everything on the page with the word “hello”.
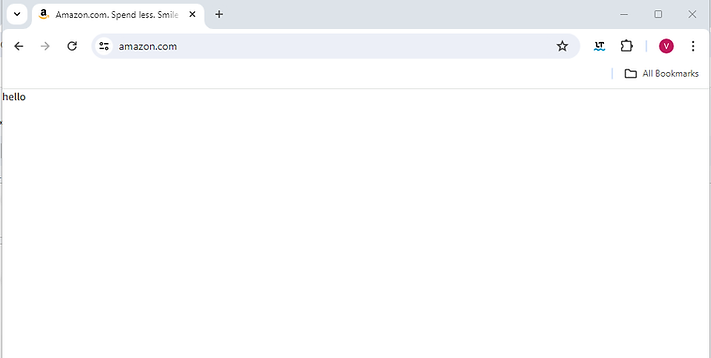
Numbers and Math
JavaScript also supports math operations. For example, addition is represented by the plus sign(+), subtraction by the minus sign (-), multiplication by the asterisk (*), and division by theforward slash (/). These symbols are also called “operators”.For example:
Syntax rules for numbers and math in JS
In JavaScript, when you're performing mathematical operations or working with numerical values, the syntax closely mirrors traditional mathematical notation. This means you can use familiar symbols and expressions directly in your code. You can also use parentheses to group operations and control the order of calculation, just like you would in a mathematical equation.
Besides that, JS not only supports integers but also decimals, so you can perform calculations like this.
Order of operations.
Multiplication (*) and division (/) have the same priority, so if both appear in the same calculation. You need to calculate from left to right. In JavaScript, both multiplication and division share the same level of operator precedence. This means that if an expression contains both multiplication and division operations, they are evaluated from left to right, regardless of which operation appears first.
For example, in the expression 10 / 2 * 3, the division operation 10 / 2 would be performed first, resulting in 5. Then, the multiplication operation 5 * 3 would be performed, giving a final result of 15. This left-to-right evaluation also applies when an expression contains multiple multiplication and division operations. For instance, in the expression 12 * 4 / 2 * 3, the operations would be evaluated as follows:
-
12 * 4 = 48
-
48 / 2 = 24
-
24 * 3 = 72
Therefore, the final result of the expression would be 72.
On the other hand, addition(+) and subtraction (-) also have the same priority, so if both appear in the same calculation. You need to calculate from left to right as well, regardless of whether the addition or subtraction comes first. This left-to-right evaluation is crucial to ensure consistent and predictable results in your JavaScript code.
For example, consider the expression 5 - 3 + 2.
If addition were given a higher priority, the expression would be incorrectly evaluated as 5 - (3 + 2), resulting in 0. However, due to the left-to-right rule, the expression is correctly evaluated as (5 - 3) + 2, resulting in 4. Remember that this left-to-right rule only applies when addition and subtraction operators are at the same level of priority. If other operators with higher or lower precedence are present, such as multiplication (*) or division (/), those operators will be evaluated according to their own precedence rules. Moreover, keep in mind that parentheses can be used to override the default operator precedence and control the order in which operations are evaluated. Any expression within parentheses is evaluated first, before the surrounding operations.
How to round a number
In programming, numbers can either integers (1,2,3,4…) or floats (1.1, 2.2, 3.3,…) and computers have issues working with floats.
Computers store and process data using the binary system, whereas humans use the decimal system. Consequently, when performing calculations with decimals (floats), the outcome might contain an unexpectedly large number of digits.
A good practice is to work with whole numbers. For example, if you want to calculate the sum of $20.95 and $7.99 you need to calculate the in cents and then divided into 100.
It is also possible to round a number by using the Math.round() function.
Strings
In JavaScript, a string is a fundamental data type used to represent textual data. Strings are sequences of characters, which can include letters, numbers, symbols, punctuation marks, and even whitespace. To create a string in JavaScript, the characters must be enclosed within quotation marks.
JavaScript provides flexibility by allowing the use of both single quotes (' ') and double quotes (" ") to delimit strings. This means that both 'hello' and "hello" are valid string in JavaScript. The choice between single and double quotes is often a matter of personal preference or stylistic consistency within a codebase. However, there are situations where one type of quote might be more convenient. For example, if a string contains an apostrophe ('), it's easier to enclose it in double quotes to avoid escaping the apostrophe within single quotes.
Strings in JavaScript are immutable, which means that once a string is created, its value cannot be changed. If you need to modify a string, you'll need to create a new string with the desired changes.
Strings can also be connected to one another on what’s called concatenation. In JavaScript, the process of joining or linking two or more strings together is known as concatenation. This is a fundamental operation used extensively in various programming scenarios where you need to combine textual data.
Illustrative Example
Let's consider a simple example to demonstrate the concept of concatenation in JavaScript.
In this code snippet, we have two string variables, 'greeting' and 'name'. We then create a new string variable 'message' by concatenating 'greeting', a space character " ", and 'name'. The plus sign (+) is the concatenation operator in JavaScript. Finally, we use the 'console.log' function to display the concatenated string 'message', which results in the output "Hello Alice".
Key Points
-
Concatenation is a common technique for dynamically building strings based on changing data.
-
The plus sign (+) operator is the primary way to concatenate strings in JavaScript.
-
You can concatenate strings with other data types; they will be implicitly converted to strings.
Type Coercion in JavaScript
Type coercion is the process of automatic or implicit conversion of values from one data type to another. This occurs in JavaScript when operations are performed on values of different data types. JavaScript is a weakly typed language, meaning it allows these conversions to happen without explicit casting.
Examples of Type Coercion:
-
String Concatenation: When using the '+' operator with a string and a number, the number is coerced into a string. For example, "5" + 3 results in "53".
-
Comparison Operators: When comparing values of different types using operators like '==', '>', '<', etc., type coercion might occur. For example, "5" == 5 evaluates to true because the string "5" is coerced into a number before the comparison.
-
Arithmetic Operations: When performing arithmetic operations with a number and a string that can be parsed as a number, the string is coerced into a number. For example, 5 - "3" results in 2.
-
Boolean Context: In boolean contexts, such as if statements and loops, values are coerced into booleans. Falsy values include 0, null, undefined, the empty string "", and NaN. All other values are truthy.
Implicit vs. Explicit Type Coercion
-
Implicit Type Coercion: This occurs automatically by JavaScript without the developer explicitly specifying the conversion.
-
Explicit Type Coercion: This is done by the developer using built-in functions like Number(), String(), Boolean(), or parseInt().
Benefits and Drawbacks of Type Coercion
-
Benefits:
-
Can make code more concise and readable in some cases.
-
Provides flexibility when working with different data types.
-
-
Drawbacks:
-
Can lead to unexpected results and bugs if not understood properly.
-
Can make code harder to debug and maintain.
-
Best Practices
-
Understand the Rules: Learn the rules of type coercion in JavaScript to avoid unexpected outcomes.
-
Use Explicit Type Coercion: When in doubt, use explicit type coercion to make your code intentions clear.
-
Be Consistent: Follow consistent coding practices and avoid mixing implicit and explicit type coercion unnecessarily.
-
Use Strict Equality: Use the '===' operator for strict equality comparison, which checks both value and type, to prevent type coercion issues.
3 Ways of creating a string
In JavaScript, strings, which represent sequences of characters, can be created using several methods. The most common approach involves enclosing the desired text within either single quotes (' ') or double quotes (" "). This flexibility allows developers to choose the quotation mark style that best suits their code or avoids conflicts with embedded characters.
In scenarios where a string needs to contain quotation marks that match the enclosing ones, the backslash (\) character can be used as an escape character. This means that the subsequent character should be interpreted literally, rather than as a string delimiter. For example, the string ‘I’m learning JS’ can be correctly represented as ‘I\’m learning JS’.
In JavaScript, strings are sequences of characters used to represent text. While single and double quotes are commonly used to define strings, backticks (``) offer a more versatile approach. These backticks are not the same as single quotes and provide additional functionalities beyond delimiting strings.
The string created using backticks are also called template strings, and they preserve any whitespace and line breaks within the backticks, maintaining the formatting of the string as it is written. This can be useful for creating multi-line strings or maintaining the indentation of code snippets.
Template strings offer a powerful mechanism for embedding expressions and variables directly into strings. This dynamic capability streamlines the creation of formatted text and simplifies the concatenation of values within strings.
Beyond basic string interpolation, template strings introduce enhanced features such as multi-line support, eliminating the need for cumbersome newline characters. This improves code readability and maintainability, particularly when dealing with lengthy or complex string structures.
Furthermore, template strings enable the incorporation of tagged templates, a sophisticated technique for parsing and manipulating template content before it is evaluated. This advanced functionality opens doors for custom formatting, data validation, and other specialized string processing operations.
Overall, template strings represent a valuable addition to the JavaScript language, offering a versatile and efficient solution for constructing dynamic and expressive strings within your code.
Interpolation
In JS interpolation is the process of adding a value directly through a string by using ${ }In JavaScript, string interpolation is the process of embedding expressions directly into a string literal using template literals. This is achieved by enclosing the expression within ${} within a string enclosed by backticks (``).
Benefits of String Interpolation
-
Readability: String interpolation makes code more concise and easier to read compared to string concatenation using the '+' operator.
-
Flexibility: It allows for the direct inclusion of variables, expressions, and even function calls within a string.
Key Points
-
Template literals are delimited by backticks (``).
-
Expressions to be evaluated are placed within ${}.
-
String interpolation is evaluated at runtime.
-
Any valid JavaScript expression can be used within ${}.
HTML, CSS & JavaScript
HTML, which stands for Hyper Text Markup Language, is the standard language used to create web pages. It is a fundamental building block of the World Wide Web and provides the structure and content for websites.
-
Hyper Text: Refers to the links that connect web pages, allowing users to navigate between them.
-
Markup Language: Uses tags to define elements within a document, such as headings, paragraphs, images, and links.
HTML works in conjunction with other web technologies like:
-
CSS (Cascading Style Sheets): Controls the visual presentation and layout of web pages.
-
JavaScript: Adds interactivity and dynamic behavior to websites.
Together, these technologies enable the creation of rich and engaging web experiences.
Variables
A variable is a container in which a valued can be stored. In computer programming, a variable acts as a symbolic name or identifier that represents a specific memory location where data can be stored and subsequently retrieved. This data can be of various types, such as numbers (integers or decimals), text (strings), or more complex data structures. The value assigned to a variable can be modified during the execution of a program, allowing for dynamic and flexible data manipulation.
Think of a variable as a labeled box where you can put different items. The label on the box is the variable's name, and the item inside is the value stored in the variable. You can change the item in the box whenever you need to, just like you can update the value of a variable in a program.
For example:
In the following example, the word “let” creates a new variable under the name “variable1” and then a value such as “3” can be stored in the variable.
Syntax Rules for Variables
-
"let" is used to declare variables. A variable is essentially a named container for storing data that you can use and manipulate throughout your code. When you create a variable with "let", you're reserving a spot in the computer's memory to hold a value.
-
“variable1” is the name of the variable.
-
“=” is the assignment operator. It is used to assign a value to a variable.
-
“3” is the value or the variable.
-
“;” is used to terminate statements.
Syntax Rules for Variables
Choosing appropriate names for your variables is crucial for writing readable and maintainable code. However, there are certain restrictions you must adhere to:
-
Reserved Words: You cannot use JavaScript reserved words as variable names. These are words that have special meanings within the language itself, such as "let", "var", "const", "if", "else", and many others.
-
No Starting with a Number: Variable names cannot begin with a number. They can, however, contain numbers as long as they don't start with one. For example, "myVariable1" is acceptable, but "1myVariable" is not.
-
Special Characters: Most special characters are not allowed in variable names. The only exceptions are the dollar sign ($) and the underscore (_). These can be used anywhere in the variable name, including at the beginning.
Best Practices for Variable Naming
While following the rules above will ensure your code is valid, adhering to some best practices will make your code more understandable and easier to work with:
-
Descriptive Names: Use descriptive names that reflect the purpose of the variable. For example, if you're storing a user's age, "userAge" is a better choice than "x".
-
Camel Case: A common naming convention is to use camel case for variable names. This means the first word is lowercase, and subsequent words start with a capital letter. For example, "firstName", "lastName".
-
Avoid Single-Letter Names: While single-letter names are sometimes used for loop counters or generic variables, they can be confusing in other contexts.
-
Consistency: Be consistent in your naming conventions throughout your code.
Re-assigning a value of a variable
To reassign a value to a variable, you need to first type the name of the variable, followed by the assigning operator, followed by the new value and a semicolon. In JavaScript, reassigning a value to an existing variable is a fundamental operation. This process involves updating the variable's current value with a new one. To achieve this, you'll need to follow a specific syntax:
1. Variable Name: Begin by typing the name of the variable that you want to modify. This should be the same identifier that you used when you initially declared the variable.
2. Assignment Operator: Next, use the assignment operator (`=`). This operator assigns the value on its right side to the variable on its left side.
3. New Value: After the assignment operator, provide the new value that you want to assign to the variable. This value can be of any valid data type in JavaScript, such as a number, string, boolean, object, or even another variable.
4. Semicolon: Finally, conclude the statement with a semicolon (`;`). This semicolon indicates the end of the reassignment statement.
Example:
Let's say you have a variable named “variable1” that currently holds the value 10. To reassign its value to 20, you would write:
Naming conventions
Naming conventions in JavaScript help make code more readable, maintainable, and consistent. Here are the most famous naming conventions:
1. camelCase (Common for variables, functions, and object properties)
-
First word is lowercase, subsequent words are capitalized.
-
Used in JavaScript for variable and function names.
2. PascalCase (Common for class names and constructors)
-
Every word starts with an uppercase letter.
-
Used for class names and constructor functions.
3. snake_case (Less common in JavaScript, used in some constants)
-
Words are lowercase and separated by underscores.
-
Mostly seen in database field names or some older JavaScript code.
4. kebab-case (Used in file names, URLs, and CSS classes)
-
Words are lowercase and separated by hyphens.
-
Not valid for variable names in JavaScript, but common in CSS and URLs.
5. UPPER_CASE (Common for constants and environment variables)
-
All letters are uppercase, words separated by underscores.
-
Used for global constants.
Reassignment shortcuts
Reassignment shortcuts in JavaScript are shorthand operators that combine an arithmetic, bitwise, or string operation with an assignment (=). These shortcuts help write more concise and readable code.
Common Reassignment Shortcuts:
Addition assignment (+=): it calculates the sum of the left and right operands and assigns the result to the left operand.
Subtraction assignment (-=): it subtracts the value of the left operand from the right operand and assigns the result to the left operand.
Multiplication assignment (*=): Multiplies the left operand by the right operand and assigns the result to the left operand.
Division assignment (/=): Divides the left operand by the right operand and assigns the result to the left operand.
Increment assignment (++;): It increases the value of a variable by 1.
-
Decrement assignment (--;): It decreases the value of a variable by 1.
How are variables declared?
In JavaScript, declaring variables is essential for storing and managing data within your code. The keywords “var”, “let”, and “const” are used for variable declaration, each with its distinct behavior and scope.
-
var: The oldest keyword for variable declaration in JavaScript. Variables declared with var have function scope, meaning they are accessible throughout the function in which they are declared. If declared outside a function, they become global variables and are accessible from anywhere in the code. However, using global variables is generally discouraged due to potential naming conflicts and maintainability issues.
-
let: Introduced in ES6 (ECMAScript 2015), let provides block scope for variables. A block is defined by curly braces {}, such as within a function, loop, or conditional statement. Variables declared with let are only accessible within the block in which they are declared, promoting better code organization and preventing unintended variable shadowing.
-
const: Also introduced in ES6, const is used to declare constants, which are variables whose values cannot be reassigned after their initial declaration. Constants also have block scope, similar to let. Using const for values that should not change helps improve code readability and prevents accidental modifications.
Key Differences and Best Practices:
-
Scope: var has function scope, while let and const have block scope.
-
Reassignment: var and let allow reassignment of values, while const does not.
-
Hoisting: Variables declared with var are hoisted to the top of their scope, meaning they can be used before their declaration (although with an undefined value). let and const are also hoisted but cannot be used before their declaration.
-
Best Practices:
-
Use const by default for values that should not change.
-
Use let for variables that need to be reassigned within their block scope.
-
Avoid using var due to its potential for scope-related issues.
-
Always declare variables before using them to prevent unexpected behavior.
-
By understanding the differences between var, let, and const and following best practices for variable declaration, you can write cleaner, more maintainable, and error-resistant JavaScript code.
Boleans and ifs-statements
In JavaScript, Boolean data types are fundamental elements that represent logical values. They can only have one of two possible states: true or false. These values are essential for controlling program flow, making decisions, and evaluating conditions. Boolean values are often used in conditional statements like "if" statements, loops, and comparison operations. They allow you to execute specific code blocks based on whether a given condition is true or false.
Comparison Operators in JavaScript
In JavaScript, comparison operators are used to compare values and return a boolean result (true or false). They are essential for controlling the flow of your code, particularly within conditional statements like if and switch. Here's a breakdown of the comparison operators available in JavaScript:
-
Equal (==): This operator checks if two values are equal, performing type coercion if necessary. For example, 3 == "3" would return true because JavaScript converts the string "3" to a number before the comparison.
-
Strict Equal (===): This operator checks if two values are strictly equal, meaning both their values and data types must match. So, 3 === "3" would return false because the number 3 and the string "3" have different data types.
-
Not Equal (!=): This operator checks if two values are not equal, performing type coercion if necessary.
-
Strict Not Equal (!==): This operator checks if two values are strictly not equal, meaning either their values or data types (or both) must differ.
-
Greater Than (>): This operator checks if the value on the left is greater than the value on the right.
-
Less Than (<): This operator checks if the value on the left is less than the value on the right.
-
Greater Than or Equal To (>=): This operator checks if the value on the left is greater than or equal to the value on the right.
-
Less Than or Equal To (<=): This operator checks if the value on the left is less than or equal to the value on the right.
If-Statement in JavaScript
Conditional statements allow us to create different blocks of code and execute the one that meets the specified condition.
Syntax and Structure
The if-statement in JavaScript is used to execute code conditionally, based on the evaluation of an expression. The basic structure is as follows:
-
The condition is an expression that evaluates to either true or false.
-
The code block within the curly braces `{}` is executed only if the condition evaluates to true.
-
If the condition evaluates to false, the code block is skipped.
Example:
In this example, the code within the if-statement will only run if the value of the 'age' variable is 18 or greater.
Else Clause
The if-statement can be extended with an else clause to provide an alternative code block to execute if the condition is false:
Example:
Else If Clause
For multiple conditions, you can use the else if-clause to chain additional checks:
Example:
Nested If-Statements
If-statements can be nested within other if-statements to create more complex conditional logic:
Important Considerations
-
Always use parentheses `()` around the condition expression in an if-statement.
-
Use curly braces `{}` to define the code block for the if-statement, even if it contains only a single statement. This improves code readability and prevents errors.
-
Be mindful of the indentation within if-statements and their code blocks. Proper indentation enhances code readability and makes it easier to understand the logic flow.
-
The if-statement is a fundamental control flow structure in JavaScript and is essential for creating dynamic and interactive web applications.
&& and || in JavaScript
In JavaScript, "&&" is the logical AND operator. It is used to combine two or more conditions or expressions, and it returns true if all the conditions are true. If any of the conditions is false, it returns false.
Here’s how it works in practice:
Simple Boolean logic
The || operator in JavaScript is the logical OR operator. It is used to combine two or more conditions or expressions, and it returns true if at least one of the conditions is true. If all conditions are false, it returns false.
How it works:
-
If at least one of the conditions is true, it will return true.
-
If all conditions are false, it will return false.
Basic boolean logic
Truthy and Falsy Values
In JavaScript, truthy and falsy values determine how expressions evaluate in a Boolean context, such as conditions in if statements.
Falsy Values
A value is falsy if it is treated as false when evaluated in a Boolean context. There are only seven falsy values in JavaScript:
-
false (the Boolean false)
-
0 (the number zero)
-
-0 (negative zero)
-
"" or '' (empty string)
-
null (empty or unknown value)
-
undefined (a variable that has been declared but not assigned a value)
-
NaN (Not-a-Number)
All other values are truthy.
Truthy Values
A value is truthy if it is not in the list of falsy values. Some common examples include:
-
Any nonzero number (1, -1, 3.14, etc.)
-
Any non-empty string ("hello", 'false', "0", etc.)
-
Arrays ([]) and objects ({}) (even if empty)
-
The special value "false" (because it is a non-empty string)
-
The value Infinity and -Infinity
Understanding truthy and falsy values helps when using logical operators (&&, ||, !) and writing concise conditional statements.
Shortcuts for if-statements
In JavaScript, you can use shortcuts to write more concise if statements. Here are some common techniques:
1. Ternary Operator (? :) – Shorthand for if...else
Instead of:
Use:
Functions
In JavaScript, a function serves as a reusable block of code designed to perform a specific task. It encapsulates a set of instructions that can be invoked repeatedly throughout your program, promoting code organization, maintainability, and efficiency. By defining a function, you give a name to a series of statements, allowing you to execute those statements simply by calling the function's name.
This eliminates the need to duplicate code whenever you want to perform the same action, saving time and effort while reducing the potential for errors. Functions can also accept input parameters (arguments) and return values, making them adaptable to different scenarios and enabling them to produce results that can be used elsewhere in your code.
Syntax Rules for Functions in JavaScript
1. Function Declaration
Rules:
-
The keyword function is required.
-
The function name must be a valid identifier (letters, numbers, _, $, but cannot start with a number).
-
Parentheses () contain parameters (can be empty).
-
The function body {} contains the code.
-
The return statement (optional) specifies the function's output.